In this tutorial, we'll explore how to implement file uploads using React on the frontend and Node.js with Multer on the backend. Uploading files is a common requirement for many web applications, whether it's images, documents, or any other type of file. By the end of this tutorial, you'll have a complete understanding of how to build a file upload feature that allows users to seamlessly upload and store files on your server.
YouTube Video Link:
Uploading Files With React + Node
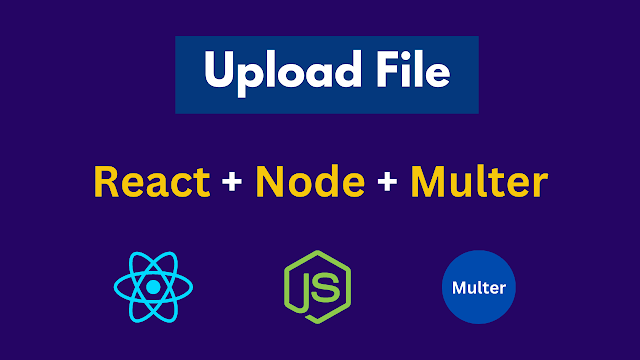
Let's Start:
Front-End code for selecting file:
install axios on your front-end app
npm install axios
import React, { useState } from "react"
import axios from 'axios'
function App() {
const [file, setFile] = useState()
const upload = () => {
const formData = new FormData()
formData.append('file', file)
axios.post('http://localhost:3001/upload',formData )
.then( res => {})
.catch(er => console.log(er))
}
return (
<div>
<input type="file" onChange={(e) => setFile(e.target.files[0])}/>
<button type="button" onClick={upload}>Upload</button>
</div>
)
}
export default App;
Server Side code:
When you created your app install the following packages
npm install express cors multer
const express = require('express')
const cors = require('cors')
const multer = require('multer')
const app = express()
app.use(cors())
app.use(express.json())
const storage = multer.diskStorage({
destination: function(req, file, cb) {
return cb(null, "./public/Images")
},
filename: function (req, file, cb) {
return cb(null, `${Date.now()}_${file.originalname}`)
}
})
const upload = multer({storage})
app.post('/upload', upload.single('file'), (req, res) => {
console.log(req.body)
console.log(req.file)
})
app.listen(3001, () => {
console.log("Server is running")
})
0 Comments